How to Solve a Complex SQL and NoSQL Database Assignment
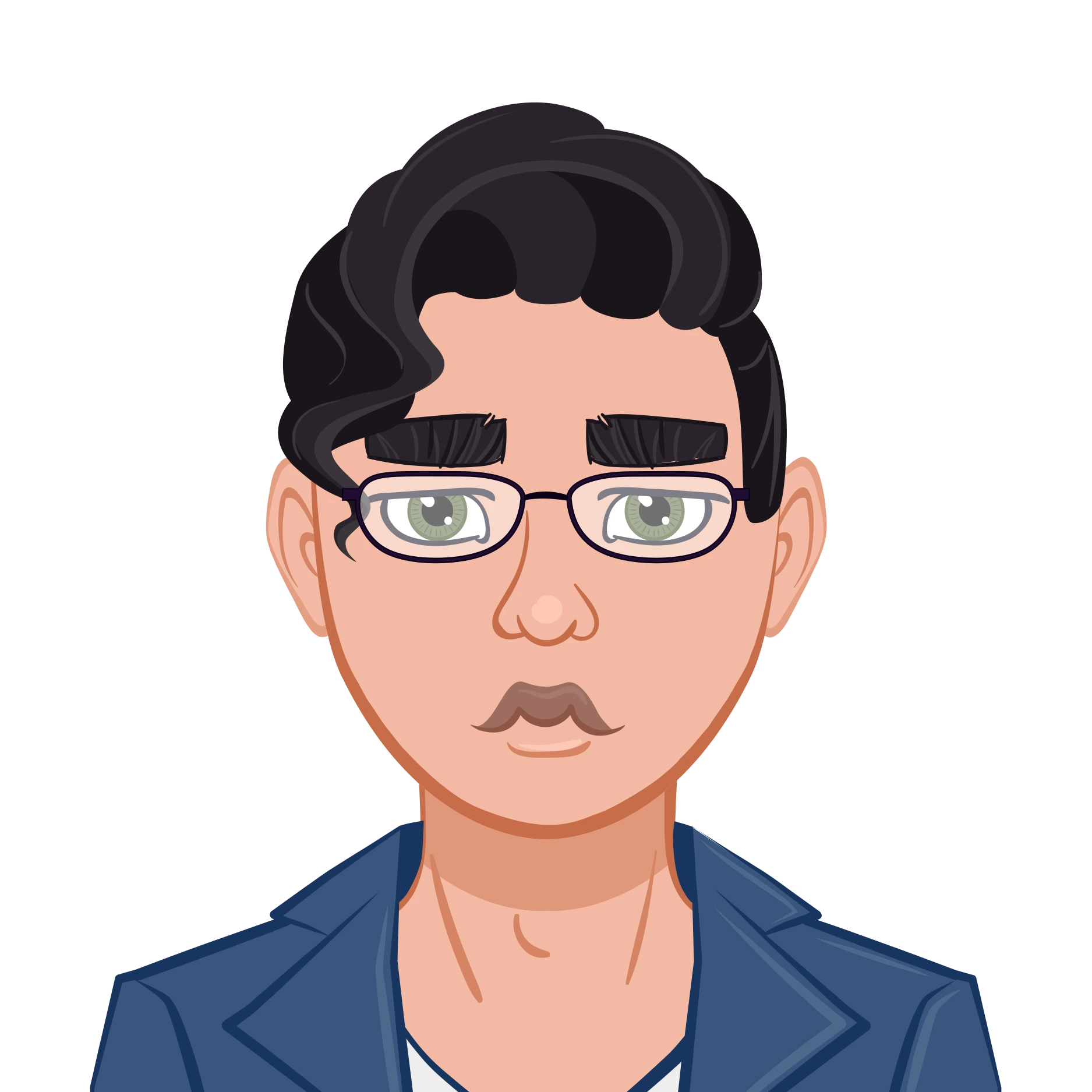
Solving a database assignment requires a structured approach, as it involves understanding database design, data management, and query execution. Whether you are working on SQL or NoSQL databases, the key to success is following a well-defined methodology. This guide provides essential steps to efficiently tackle database assignments, ensuring accuracy and effectiveness. For students looking for database homework help, it is important to begin by thoroughly understanding the assignment requirements and gathering all necessary resources. A well-planned approach can make tasks such as schema design, data normalization, and query formulation more manageable. Furthermore, leveraging cloud-based services like Microsoft Azure enhances scalability and accessibility. Students often seek help with SQL homework to navigate complex queries, indexing, and transaction management, which are crucial for handling large datasets. Implementing best practices, such as writing optimized queries and ensuring data security, can significantly improve the quality of the assignment. Additionally, debugging techniques and thorough documentation play a crucial role in achieving precise results. By following a structured approach and utilizing expert guidance when needed, students can successfully complete their database assignments while gaining valuable real-world skills.
Understanding the Assignment Requirements
Understanding the assignment requirements is the first step in solving a database assignment successfully. It involves analyzing the problem statement, identifying the expected outcomes, and reviewing any provided guidelines, datasets, or scripts. Carefully assessing the specifications helps in defining the scope of work, selecting appropriate tools, and ensuring compliance with evaluation criteria. Additionally, understanding database constraints, relationships, and business logic is essential for developing an effective solution. By clarifying all aspects beforehand, students can avoid unnecessary errors and streamline their approach. Additionally, the task involves linking a Python application to manage transactions and generate reports.
Breakdown of the Assignment Objectives:
- Design and implement a SQL and NoSQL database on Microsoft Azure.
- Retrieve and modify transaction data from the central SQL database.
- Store orders and driver details in a NoSQL database.
- Generate cooking and delivery dockets.
- Summarize daily operations and write reports to SQL.
Preparing to Solve the Assignment
Before starting the actual implementation, preparation is key to executing the assignment efficiently. Begin by setting up the necessary software, such as database management systems, cloud platforms, or programming environments. Organize all reference materials, including database schema, sample data, and queries, to facilitate easy access during development. Creating a structured plan, such as breaking down tasks into manageable steps, can help in tracking progress effectively. Reviewing relevant concepts in SQL and NoSQL databases, data modeling, indexing, and query optimization will ensure a smoother execution process. Adequate preparation helps minimize delays and enhances overall productivity. To approach this assignment efficiently, follow these preparatory steps:
- Gather the necessary tools and resources: Ensure access to Microsoft Azure, Python (including libraries like pyodbc and pymongo), and SQL query editors.
- Understand the database structure: Review provided SQL scripts (Create Tables.sql and Load Data.sql) to understand data types, relationships, and schema.
- Plan the database design: Determine the structure of your NoSQL database for storing orders and drivers.
- Set up the working environment: Install and configure required software, including setting up Azure SQL and NoSQL databases.
Implementing the Database Solution
Implementing the database solution involves executing the planned design and writing necessary scripts to handle database operations. Start by creating the database schema, defining tables, relationships, and constraints according to best practices. Populate the database with sample data to test queries and validate the integrity of the structure. Write optimized SQL or NoSQL queries to perform transactions, retrieve records, and generate reports. If using Python or another programming language, ensure seamless integration between the application and the database. Debugging errors, refining queries, and validating outputs are essential steps before final submission. A well-implemented database solution ensures accuracy, efficiency, and scalability in handling real-world scenarios.
1. Setting Up SQL and NoSQL Databases
- SQL Database (Microsoft Azure): Create a table for storing summary reports.
- NoSQL Database (MongoDB on Azure): Design collections for order storage and driver details.
Database Schema Design:
SQL Table (Summary Table in Azure SQL Database):
CREATE TABLE daily_summary (
store_id INT,
total_orders INT,
total_sales DECIMAL(10,2),
most_popular_pizza VARCHAR(255),
total_driver_commission DECIMAL(10,2),
PRIMARY KEY (store_id)
);
NoSQL Collections (MongoDB):
{
"orders": {
"order_id": "string",
"store_id": "int",
"items": [
{"pizza_name": "string", "quantity": "int"}
],
"total_price": "decimal",
"assigned_driver": "string"
},
"drivers": {
"driver_id": "string",
"name": "string",
"delivery_suburbs": ["string"],
"commission_rate": "decimal"
}
}
2. Writing a Python Program to Connect to Databases
Connect to SQL Database:
import pyodbc
conn = pyodbc.connect(
'DRIVER={SQL Server};'
'SERVER=ict320-task3a.database.windows.net;'
'DATABASE=joe-pizzeria;'
'UID=student320;'
'PWD=ICT320_student'
)
cursor = conn.cursor()
Connect to NoSQL Database (MongoDB):
from pymongo import MongoClient
client = MongoClient("mongodb+srv://username:password @cluster.mongodb.net/")
db = client["joe_pizzeria"]
orders_collection = db["orders"]
drivers_collection = db["drivers"]
3. Processing Orders and Generating Reports
Download transactions from SQL and store them in NoSQL:
cursor.execute("SELECT * FROM orders WHERE store_id=1")
orders = cursor.fetchall()
for order in orders:
orders_collection.insert_one({
"order_id": order.order_id,
"store_id": order.store_id,
"items": order.items,
"total_price": order.total_price,
"assigned_driver": None
})
Assign drivers and generate delivery dockets:
drivers = list(drivers_collection.find())
for order in orders_collection.find():
assigned_driver = drivers[order["order_id"] % len(drivers)]
orders_collection.update_one(
{"order_id": order["order_id"]},
{"$set": {"assigned_driver": assigned_driver["name"]}}
)
Generate a daily summary:
cursor.execute(
"INSERT INTO daily_summary (store_id, total_orders, total_sales, most_popular_pizza, total_driver_commission) "
"VALUES (?, ?, ?, ?, ?)",
(1, 100, 2500, "Pepperoni Pizza", 250)
)
conn.commit()
Best Practices for Completing the Assignment
Adopting best practices while working on a database assignment significantly improves its quality and efficiency. Start by maintaining a well-structured approach to database design, ensuring tables and relationships follow normalization principles for optimized performance. Writing clear, concise, and well-documented code helps in future modifications and debugging. Security should be a priority—implement access controls, encryption, and backup strategies to protect sensitive data. Use indexing and query optimization techniques to improve database performance, reducing execution time for large datasets. Regularly testing and validating database queries prevents errors and ensures consistency. Debugging tools and logging mechanisms can help track issues and provide insights into performance improvements. When integrating databases with applications, ensure efficient connection handling and transaction management to prevent data corruption. Following best practices in coding standards, error handling, and performance tuning can elevate the quality of the assignment. Additionally, seeking feedback from peers or mentors and using online resources for troubleshooting can enhance learning and refine the final implementation.
1. Document Your Work
- Clearly explain database design decisions.
- Include comments in Python scripts for better readability.
- Provide justifications for schema structures and data storage formats.
2. Testing and Debugging
- Run small test cases before handling large datasets.
- Log database interactions to troubleshoot potential errors.
- Validate SQL queries before executing them to prevent data loss.
3. Future Improvements and Security Considerations
- Implement authentication for database access.
- Optimize queries for better performance.
- Store sensitive information securely using environment variables.
- Consider deploying the application on a cloud-based server for scalability.
Conclusion
Completing a database assignment requires careful planning, structured execution, and adherence to best practices. Understanding the requirements, preparing a clear implementation plan, and executing database operations efficiently contribute to the success of the project. Leveraging SQL and NoSQL solutions appropriately while ensuring security, performance optimization, and documentation leads to a robust and scalable database design. Debugging and testing throughout the development process minimize errors and enhance the accuracy of the final solution. Additionally, maintaining a professional approach, including clear documentation and following coding standards, adds value to the assignment. Students seeking database homework help can benefit from expert guidance to refine their skills and ensure successful completion of their projects. By implementing these strategies, students can not only complete their assignments effectively but also gain valuable knowledge and experience in database management. Applying these principles in real-world scenarios enhances problem-solving skills, making database assignments a stepping stone towards professional expertise.