How to Approach and Solve SQL-Based Database Assignments
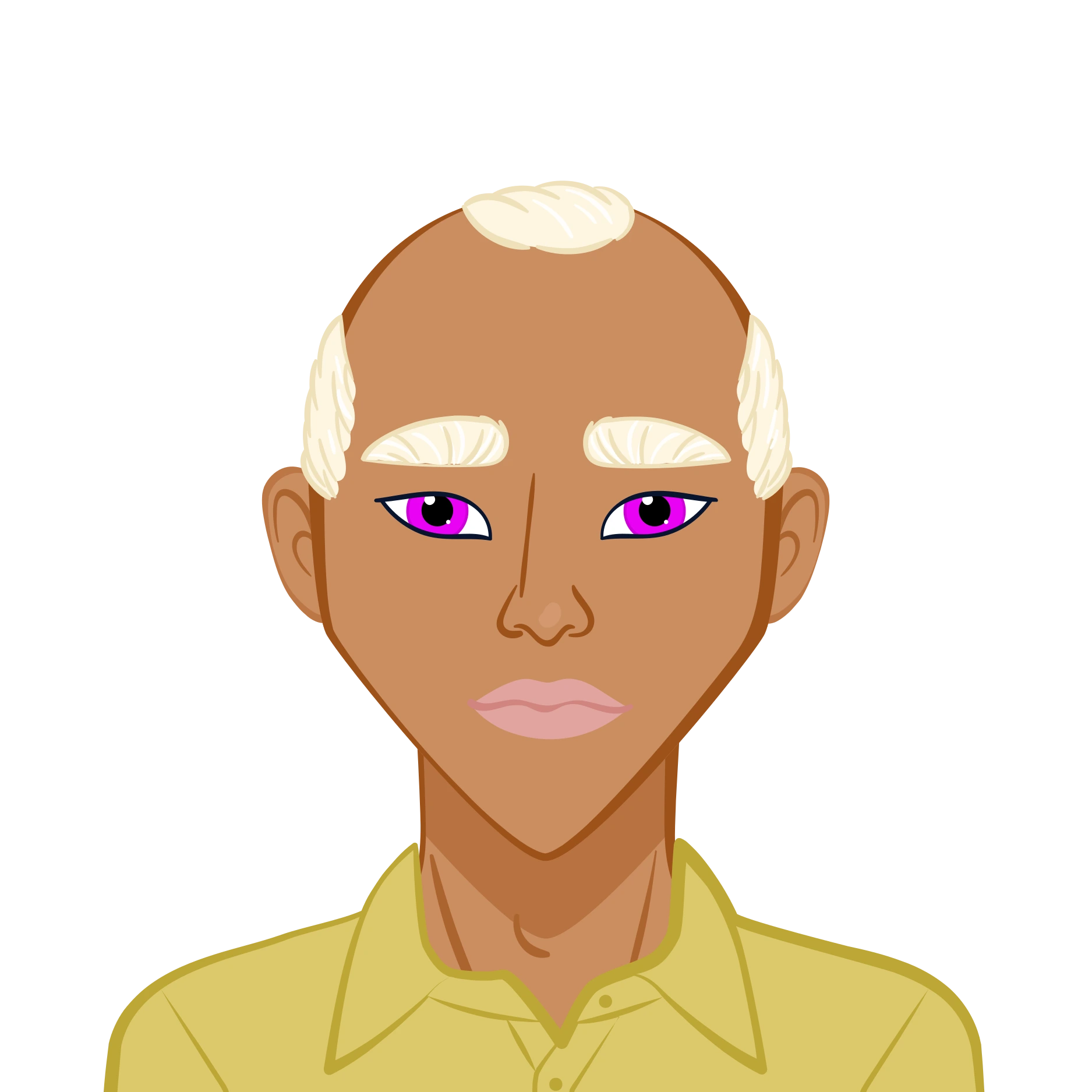
Struggling with complex database assignments? Whether you're working on SQL queries, database design, or data manipulation, having a structured approach is key to success. This guide offers database homework help by breaking down essential steps to efficiently tackle assignments. From understanding requirements and setting up your database environment to writing optimized queries and debugging errors, you’ll gain insights into best practices that enhance accuracy and efficiency. Whether you need help with MySQL homework or general database concepts, this guide ensures you can confidently handle tasks like updating records, retrieving specific data using SELECT, and performing advanced operations with GROUP BY and nested queries. With detailed explanations on formatting output, transaction handling, and query optimization, you'll learn how to approach assignments systematically and avoid common pitfalls. Additionally, we cover best practices such as using comments for documentation, logging outputs for tracking execution, and ensuring your final submission meets academic standards. By following this structured approach, you can not only complete your assignments efficiently but also strengthen your understanding of relational databases, SQL syntax, and data organization. Whether you're a beginner or an advanced learner, mastering database assignments requires a combination of logical thinking and technical skills, and this guide provides a comprehensive roadmap to achieving that. From simple data modifications to complex data retrieval and analysis, these step-by-step strategies will help you optimize your workflow and submit error-free assignments. With the right guidance and preparation, you can transform challenging database assignments into manageable tasks while improving your problem-solving skills in SQL.
Understanding the Assignment Requirements
Before diving into coding, carefully analyze the assignment instructions to ensure clarity on each task. Break down the requirements into smaller, manageable steps, distinguishing between data modifications, retrieval queries, and reporting tasks. Identify key constraints, required formats, and expected outputs. If working on a previously created database, ensure that all necessary tables and data exist by reviewing past work. Missing prerequisites can lead to incorrect outputs, so double-checking database integrity is crucial. Pay close attention to column names, data types, and relationships between tables to avoid common errors. Structuring the assignment in logical steps will help streamline the problem-solving process.
1. Read the Instructions Carefully
Before you start coding or writing queries, thoroughly read the assignment details. Identify the core objectives, whether it's modifying existing records, retrieving specific data, or structuring outputs in a particular format.
2. Break Down the Tasks
Database assignments often involve multiple smaller tasks. Breaking them down into logical steps can help you approach them systematically. For example, an assignment requiring updating room reservations and retrieving customer details should be separated into:
- Data modification (UPDATE, INSERT)
- Data retrieval (SELECT, GROUP BY, ORDER BY)
- Formatting and output requirements
3. Set Up Your Database Environment
Ensure you have the correct database schema set up. If the assignment builds on a previous project, ensure that the database is correctly initialized. Running necessary SQL scripts beforehand ensures that your tables, constraints, and relationships are intact.
Writing and Executing SQL Queries
Writing accurate and efficient SQL queries is the backbone of database assignments. Use SELECT, UPDATE, INSERT, DELETE, and JOIN statements effectively to manipulate and retrieve data. Always use WHERE clauses in updates and deletions to prevent unintended modifications. When retrieving data, employ ORDER BY for sorting, GROUP BY for aggregations, and HAVING to filter grouped results. Utilize aliasing for better readability and format outputs as required. Test queries incrementally to identify and correct errors before executing the entire script. Ensuring correctness at this stage minimizes debugging efforts later.
4. Using the Correct SQL Syntax
SQL statements should follow proper syntax. Here are some best practices:
- Updating records: Use UPDATE statements with WHERE clauses to avoid unintended changes.
- Inserting new data: INSERT INTO should be used with column names to maintain consistency.
- Deleting records: Be cautious when using DELETE; always include WHERE conditions to prevent accidental deletions.
5. Using SELECT for Data Retrieval
Retrieve data using the SELECT statement efficiently by applying:
- WHERE clauses to filter specific records
- ORDER BY for sorting
- GROUP BY for aggregations
- HAVING to filter grouped data
Example:
SELECT CustomerID, CustFName || ' ' || CustLName AS CustomerName, COUNT(*) AS ResCount
FROM Reservations
GROUP BY CustomerID, CustFName, CustLName
ORDER BY ResCount DESC;
6. Formatting Output Data
Many assignments require specific formatting, such as:
- Formatting phone numbers:
SELECT CustomerID, CustFName, CustLName,
'(' || SUBSTR(CustPhone, 1, 3) || ') ' ||
SUBSTR(CustPhone, 4, 3) || '-' || SUBSTR(CustPhone, 7, 4) AS FormattedPhone
FROM Customers;
- Displaying currency values:
SELECT ResNum, RoomNum, RateAmt, TO_CHAR(RateAmt, '$999.99') AS FormattedRate
FROM Reservations;
Optimizing Query Performance
Efficient query execution is essential for handling large datasets. Use indexes on frequently searched columns and avoid unnecessary SELECT * statements, which slow down performance. Optimize queries by using INNER JOINs instead of subqueries where possible, and leverage GROUP BY and HAVING wisely to prevent redundant calculations. Checking the execution plan can help identify performance bottlenecks. Additionally, ensure transactions are properly structured to maintain data integrity and consistency, especially when performing multiple updates.
7. Using Indexing and Joins Wisely
When dealing with large datasets, using indexes and proper joins ensures efficient query execution:
- Use INNER JOINs for retrieving related data from multiple tables.
- Optimize with indexes when filtering or sorting large tables.
Example:
SELECT r.ResNum, r.CheckIn, r.CheckOut, c.CustFName || ' ' || c.CustLName AS CustomerName
FROM Reservations r
JOIN Customers c ON r.CustomerID = c.CustomerID
ORDER BY r.CheckIn;
8. Using Subqueries and Nested SELECT Statements
Some assignments require nested queries to fetch aggregated results:
SELECT RoomNum, RoomType, RateAmt
FROM Rooms
WHERE RateAmt > (SELECT AVG(RateAmt) FROM Rooms);
Error Handling and Debugging
Debugging is a critical step in database assignments to ensure accurate results. Common issues include syntax errors, missing column references, constraint violations, and incorrect joins. Running queries step-by-step, using LIMIT to check partial outputs, and adding comments can help pinpoint errors. If working with MySQL, using SHOW WARNINGS and EXPLAIN can reveal issues in execution plans. When handling complex operations, wrapping statements in transactions allows rolling back in case of errors, preventing unintended data corruption. Proper debugging practices help maintain database reliability and accuracy.
9. Checking for Errors
Run SQL statements incrementally and check for:
- Syntax errors
- Missing or incorrect column references
- Constraint violations
10. Using Transaction Control
For assignments requiring multiple updates, using transactions ensures data integrity:
BEGIN;
UPDATE Reservations SET CheckOut = '2023-03-10' WHERE ResNum = 1001;
COMMIT;
Documenting Your Work
Clear documentation enhances the readability and maintainability of your SQL scripts. Always include comments explaining complex queries, specifying the purpose of each statement. Format SQL code neatly with proper indentation and spacing to improve clarity. If required, use logging techniques like the spool command to save query results for reference. Properly documented queries are easier to troubleshoot and modify in the future, making them a valuable practice in both academic and professional settings.
11. Adding Comments in SQL Scripts
Always document complex SQL statements:
-- Updating room rate for reservation 1010
UPDATE Reservations SET RateAmt = 110 WHERE ResNum = 1010;
12. Logging Outputs
Using the spool command (if applicable) helps in maintaining logs for executed queries:
SPOOL Project3_output.txt;
SET ECHO ON;
SELECT * FROM Reservations;
SPOOL OFF;
Finalizing the Assignment
Before submission, verify that all queries meet the assignment requirements and produce the expected results. Check formatting rules for phone numbers, currency values, and date formats if specified. Test queries with different inputs to confirm robustness and accuracy. Ensure transactions are committed where necessary, preventing data loss. Lastly, follow submission guidelines such as proper file naming conventions and including relevant details in comment sections. A final review ensures completeness, correctness, and adherence to best practices, leading to a high-quality database assignment.
13. Verifying and Testing Queries
Before submission, verify:
- Queries return correct and expected results
- Data integrity is maintained
- Formatting matches requirements
14. Submitting the Assignment
Follow submission guidelines such as:
- File naming conventions (e.g., Project3_abc.sql)
- Including student details in comment sections
By following these structured steps, you can efficiently approach and solve database assignments while ensuring accuracy, efficiency, and clarity in your SQL queries and database manipulations.